다형성이란 하나의 코드가 여러 자료형으로 구현되어 실행되는 것을 말한다.
다형성을 잘 활용하면,
상위클래스에서 공통 부분의 메서드를 제공하고, 하위클래스에서는 그에 기반한 추가 요소를 덧붙여 구현하면 코드 양도 줄어들고 유지보수도 편리해진다. 또 상속받은 모든 클래스를 하나의 상위 클래스로 처리할 수 있고 다형성에 의해 각 클래스의 여러가지 구현을 실행할 수 있으므로 프로그램을 쉽게 확장할 수 있다.
다시 말해서, 확장성 있고 유지보수 하기 좋은 프로그램을 개발할 수 있다.
package polymorphism;
import java.util.ArrayList;
class Animal {
public void move() {
System.out.println("동물이 움직입니다.");
}
}
class Human extends Animal {
public void move()
{
System.out.println("사람이 두 발로 걷습니다.");
}
public void readBook()
{
System.out.println("사람이 책을 읽습니다.");
}
}
class Tiger extends Animal {
public void move() {
System.out.println("호랑이가 네 발로 뜁니다.");
}
public void hunting() {
System.out.println("호랑이가 사냥을 합니다.");
}
}
class Eagle extends Animal {
public void move() {
System.out.println("독수리가 하늘을 납니다.");
}
public void flying() {
System.out.println("독수리가 날개를 쭉 펴고 멀리 날아갑니다.");
}
}
public class AnimalTest {
ArrayList<Animal> aniList = new ArrayList<Animal>();
public static void main(String[] args) {
AnimalTest aTest = new AnimalTest();
aTest.addAnimal();
System.out.println("원래 형으로 다운 캐스팅");
aTest.testCasting();
}
public void addAnimal()
{
aniList.add(new Human());
aniList.add(new Tiger());
aniList.add(new Eagle());
for(Animal ani : aniList)
{
ani.move();
}
}
public void testCasting() {
for(int i = 0; i < aniList.size(); i++)
{
Animal ani = aniList.get(i);
if(ani instanceof Human)
{
Human h = (Human)ani;
h.readBook();
}
else if(ani instanceof Tiger)
{
Tiger t = (Tiger)ani;
t.hunting();
}
else if(ani instanceof Eagle)
{
Eagle e = (Eagle)ani;
e.flying();
}
else
{
System.out.println("지원되지 않는 형식입니다.");
}
}
}
}
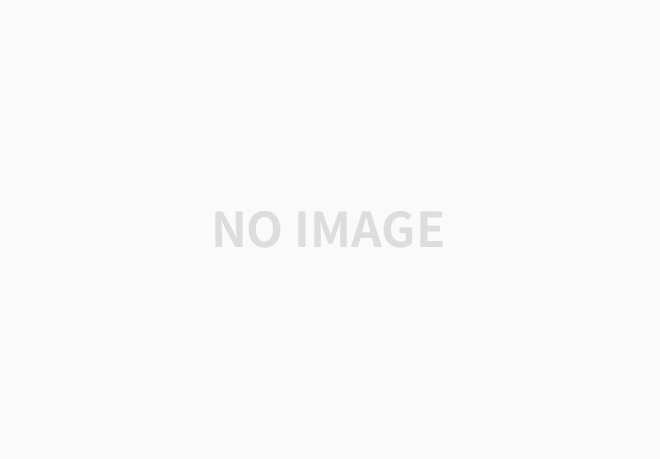
위 코드 중 addAnimal() 메서드에 ani.move() 메서드가 포함되어 있다.
ani.move() 메서드 코드 한 줄이지만 어떤 인스턴스가 들어갔느냐에 따라 move()의 실행이 달라졌는데,
이것이 다형성이다. 같은 말로, 여러 클래스를 하나의 타입(상위 클래스)로 핸들링 할 수 있다는 것이다.
만약 다형성을 사용하지 않는다면 if, else if 문을 사용해야 하고 코드가 길어지고, 유지보수가 어려워지기 때문에
다형성을 잘 활용하는 것이 좋다.
'자바 기초' 카테고리의 다른 글
자바 템플릿 메서드, final - Do it! 자바프로그래밍기초 (0) | 2022.07.22 |
---|---|
자바 추상클래스 - Do it! 자바프로그래밍기초 (0) | 2022.07.22 |
자바 메서드 오버라이딩, 클래스 형 변환 - Do it ! 자바프로그래밍기초 (0) | 2022.07.21 |
자바 상속에서 클래스 생성, super - Do it! 자바프로그래밍기초 (0) | 2022.07.20 |
자바 상속 - Do it! 자바프로그래밍 기초 (0) | 2022.07.20 |